Introduction
The AirRobeWidget Android SDK provides Circular Wardrobe widgets for your native Android application.
Integration
Requirements
- Android 5.0 (API level 21) and above
Manual Installation
Currently, we do not support any other installation methods, so for now the AirRobe SDK has to be manually integrated into your project.
Source
GitHub Release
Download the latest release source zip from GitHub and unzip into to any temporary directory outside your project directory.
Project Workspace Integration
Now that the AirRobeWidget SDK resides in your temporary directory, you can add this SDK as a module to your project with the following steps:
- Open your project on Android Studio IDE
- Import the module by going to File -> New -> Import Module...
-
Choose the library named
AirRobeWidget
inside the downloaded repo by manually inputting the path or through the file browser window showing by tapping theBrowse
icon. -
Add the imported module to your
build.gradle
dependecies.
dependencies: {
//Other dependencies are here
implementation project(':AirRobeWidget')
}
You will need to keep track of the library version manually, and when you want to upgrade to a more recent version, you will need to manually download the library and replace it.
ProGuard
If you are using ProGuard you might need to add OkHttp's rules
Getting Started
The AirRobe SDK contains UI components that can be added to your product detail screens, cart screens, and order confirmation screens. The AirRobeOptIn component is intended to be used on a single product detail screens, the AirRobeMultiOptIn component on cart screen, and the AirRobeConfirmation component on the order confirmation screen.
Widget Initialization
You are going to need your AirRobe appId
, which is used to
configure widgets and fetch up-to-date data mapping information. You can
access your AppId
here
The first step is to perform some global initialization of AirRobeWidgets:
-
appId: String
- Required - This is the id associated with your platform. -
color: String
- Optional - This sets the colour of the toggle button, dropdown caret, and links. Defaults to42abc8
-
mode: String
- Optional - The two options are.PRODUCTION
or.SANDBOX
. Defaults to.PRODUCTION
.
-
import com.airrobe.widgetsdk.airrobewidget.AirRobeWidget
import com.airrobe.widgetsdk.airrobewidget.config.AirRobeWidgetConfig
import com.airrobe.widgetsdk.airrobewidget.config.Mode
AirRobeWidget.initialize(
AirRobeWidgetConfig(
appId: "515b6ee129da",
color: "ff0000",
mode: "Mode.SANDBOX"
)
) -
import com.airrobe.widgetsdk.airrobewidget.AirRobeWidget
import com.airrobe.widgetsdk.airrobewidget.config.AirRobeWidgetConfig
import com.airrobe.widgetsdk.airrobewidget.config.Mode
AirRobeWidget.initialize(
AirRobeWidgetConfig(
appId: "...",
color: "ff0000",
mode: "Mode.PRODUCTION"
)
)
AirRobe Widgets should be added by using XML or programmatically added inside
your activity, and then configured using the initialize
function,
passing required arguments.
Opt-In View Widget Initialization
The AirRobeOptIn component should be added to your product details screen, passing arguments for the product's category and price, as well as material and brand if available. These attributes are used to fetch estimated resale price from the AirRobe Price Engine. Localisation attributes can also be provided.
This widget is used for a single item, such as on a product page. The
"Potential value" field is calculated in AirRobe's Pricing Engine from a
combination of the priceCents
, rrpCents
,
category
, brand
, and
material
arguments.
The follow arguments are required for initialization:
-
brand: String
- Optional - Describes which brand the widget belongs to. -
material: String
- Optional - Describes which material the widget belongs to. -
category: String
- Required - Describes which category the widget belongs to. -
priceCents: Double
- Required - Describes the price of the shopping item. -
originalFullPriceCents: Double
- Optional - Describes the original full price of the shopping item. -
rrpCents: Double
- Optional - Describes the Recommended Retail Price of the shopping item. -
currency: String
- Optional - Describes the currency using the ISO 4217 currency code. Default is "AUD". -
locale: String
- Optional - Describes the current locale of the device using the ISO 639 locale string. Default is "en-AU".
XML
<com.airrobe.widgetsdk.airrobewidget.widgets.AirRobeOptIn
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
Kotlin
val airRobeOptIn = findViewById<AirRobeOptIn>(R.id.opt_in_widget)
...
airRobeOptIn.initialize(
brand = "",
material = "",
category = "Hats/fancy-hats",
priceCents = "100",
originalFullPriceCents = "100",
rrpCents = "100",
currency = "AUD",
locale = "en-AU"
)
The widget will not be displayed on the page if the following conditions are met:
- The product category associated with the item hasn't been mapped to the categories in the AirRobe system. For example, your store may have a category "New Category/New Sub Category" that has not been mapped to "New Category" in the AirRobe system.
- The product is not AirRobe-eligible. The AirRobeWidget Android SDK considers a product's category, price, and department when making an eligibility determination.
Opt-In Widget Example![]() |
Multi-Opt-In View Initialization
The AirRobeMultiOptIn component should be added to your cart screen. A list of product categories should be passed as an argument during initialisation.
The follow arguments are required upon initialization:
-
items: [String]
- Required - A list of all the categories for the current items.
XML
<com.airrobe.widgetsdk.airrobewidget.widgets.AirRobeMultiOptIn
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
Kotlin
val airRobeMultiOptIn = findViewById<AirRobeMultiOptIn>(R.id.multi_opt_in_widget)
...
airRobeMultiOptIn.initialize(
arrayOf("Accessories", "Accessories")
The widget will not be displayed on the page if the following conditions are met:
- The
items
argument has an empty array as a value. - All the product categories passed in haven't been mapped to the categories in AirRobe. However, it will be displayed if any one of the categories have been mapped to AirRobe.
Multi-Opt-In Widget Example![]() |
Confirmation View Initialization
The AirRobeConfirmation component should be added to the order confirmation screen in your app. While this component should always be rendered in the activity, it will only be visible to customers if they opted in to AirRobe for their order.
This widget is shown on the order processed page. In the button it'll display
ACTIVATE YOUR AIRROBE ACCOUNT
if the email that is used doesn't
exist in the AirRobe system but if it does exist it'll show
VIEW MY AIRROBE
. The follow arguments are required upon
initialization:
-
orderId: String
- Required - The order id from the checkout. -
orderSubtotalCents: Double
- Required - Sum of line item prices including tax, less discounts, excluding any shipping costs. -
currency: String
- Optional - Describes the currency using the ISO 4217 currency code. Default is "AUD". -
email: String
- Required - The email used by the shopper.
XML
<com.airrobe.widgetsdk.airrobewidget.widgets.AirRobeConfirmation
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
Kotlin
val airRobeConfirmation = findViewById<AirRobeConfirmation>(R.id.confirmation_widget)
...
airRobeConfirmation.initialize(
orderId = "123456"
orderSubtotalCents = "11000",
currency = "AUD",
email = "test@email.com"
)
The widget will not be displayed on the page if the following conditions are met:
- If
orderId
oremail
are not passed in. - If the user doesn't opt in.
Confirmation Widget Example![]() |
Other Functionalities
Clear Cache (Opt value reset)
AirRobeWidget.resetOptedIn()
Get Order-Opt-In value
AirRobeWidget.orderOptedIn()
Example
The example project demonstrates how to include AirRobeWidget UI components.
Building & Running
Building and running the project is as simple as cloning the repository, opening AirRobeWidget
and building it.
Wrapping up
We're so excited to have you on board with us in the Circular Fashion Movement!
We're here for you
Our team is just an email away, at integration@airrobe.com. We're more than happy to answer your questions and do what we can to help make your AirRobe integration successful!
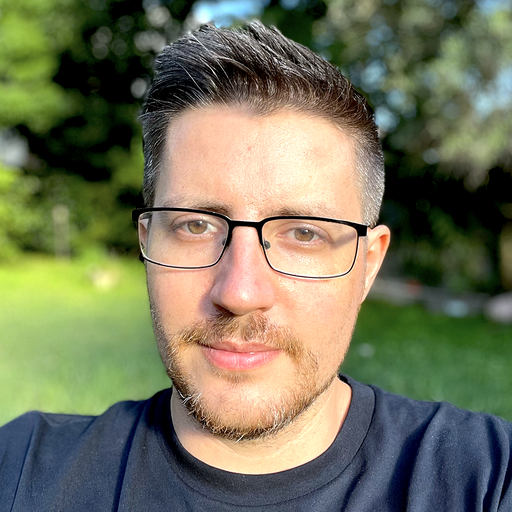
Alex
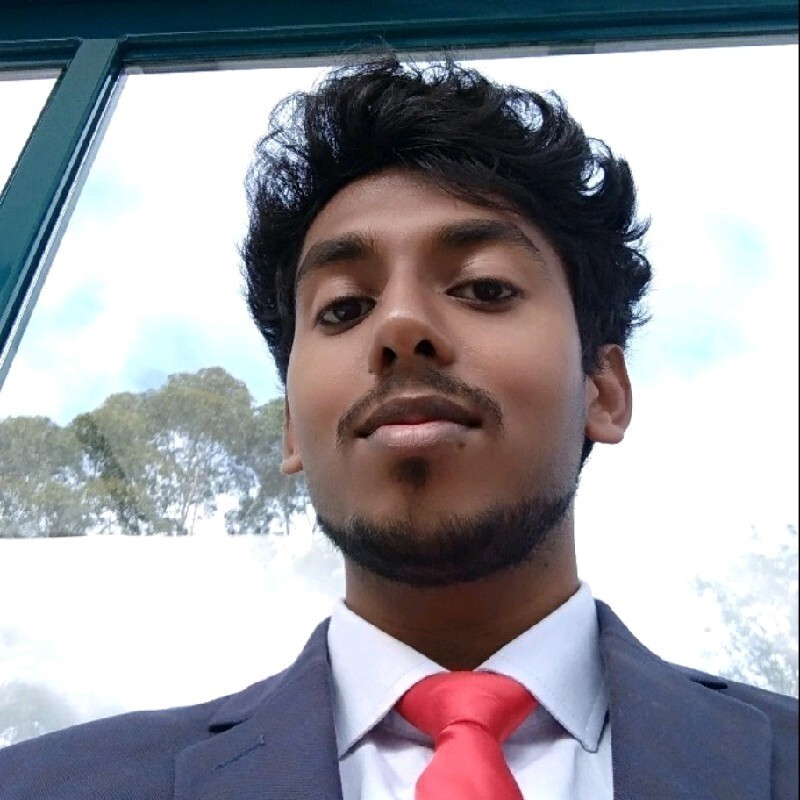
Edwin
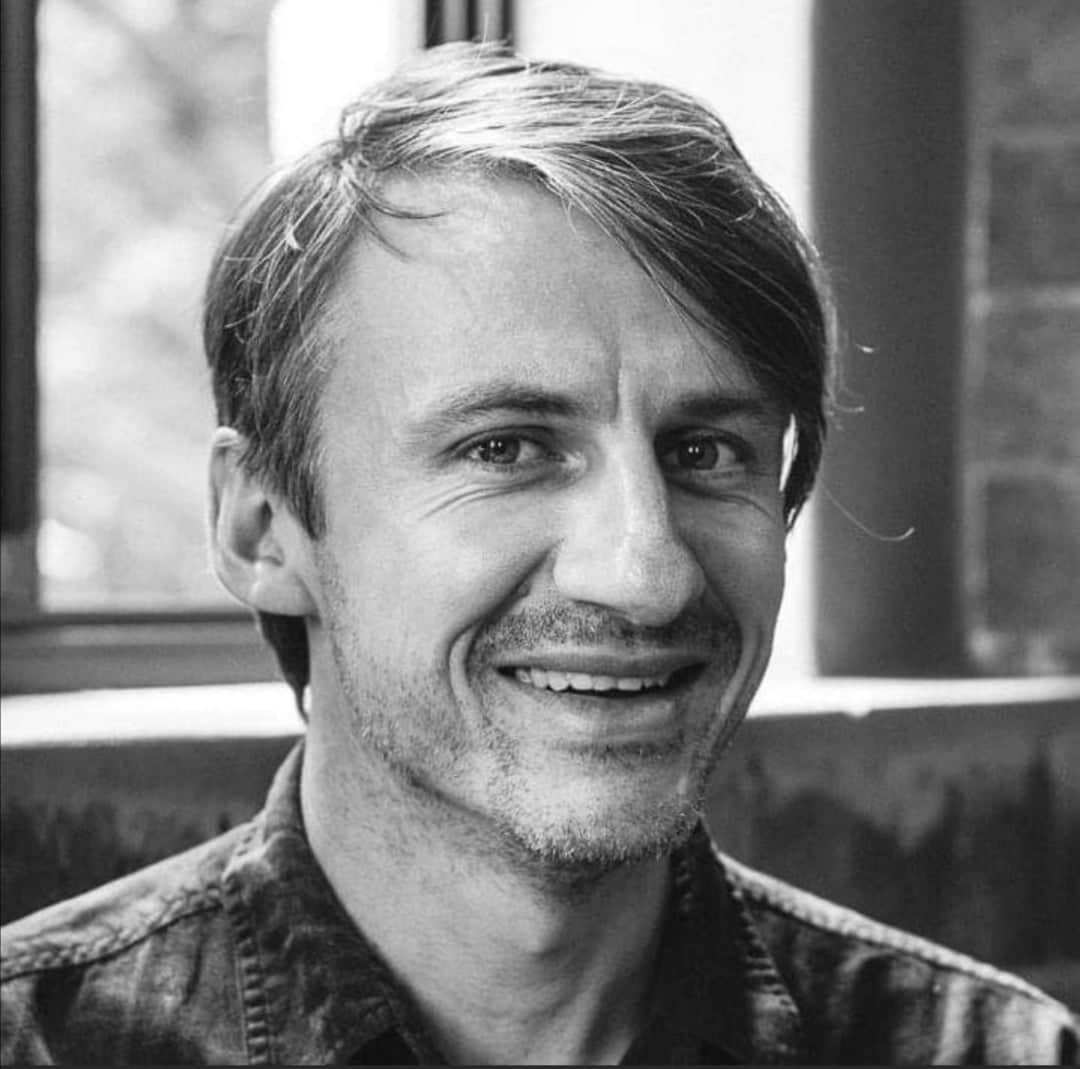
John
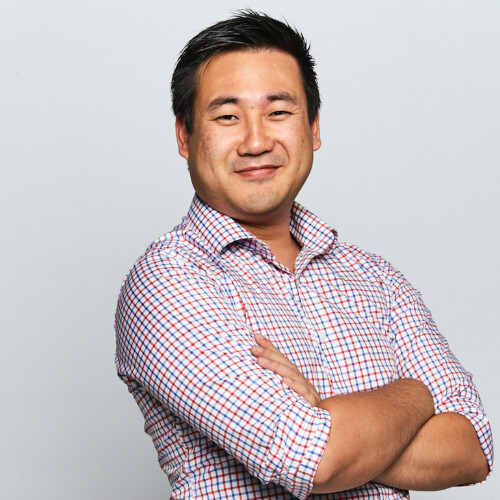
Pius
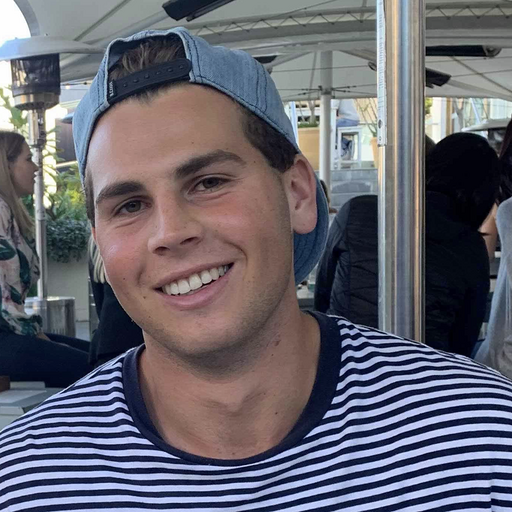